Creating a Discord bot using Python
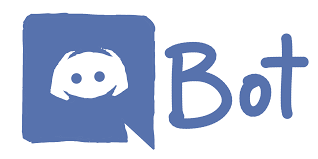
I just went through this process and thought I would document it for myself and others. In a nutshell, you register a bot app with Discord and add it to your server. Then you write the bot code which connects to this app and gives it functionality. Eventually you will want to deploy this bot to a server somewhere so it is always available.
Create your discord server
(if you don’t already have one)
In the Discord app find the servers list on the left and click the “+” to create a new server. Then select “Create Server”
Create your Discord app and bot user
You need to register your bot/app with Discord
Go to https://discordapp.com/developers/applications/me
Give it a name, and click “Create App”.
In the Bot section, click “Create Bot User”. This makes your app a Bot.
“Public Bot” option. You can leave this unchecked if you only want to use the bot in servers you admin. Check this if you want other admins to be able to add the bot to their servers.
There are lots of details you can customize, but the most important thing here is the “Client ID”. This is the unique identifier for your bot. This is the identifier that is needed to add the bot to a server. You can come back to this page any time in the future and get the client ID so just remember that it is here.
Make sure you hit “Save Changes”
Authorize the bot and add it to your server
Go to: https://discordapp.com/oauth2/authorize?client_id=XXXXXXXX&scope=bot but replace XXXXXXXX with your client ID from above. It will show you a list of servers you admin. Choose the server that you would like to add the bot user to.
Great! now your bot user is in a server. You just need to run some code on a server somewhere to receive and process commands. You can run this code anywhere and use several different languages. In my case, I used Python and run the code on my laptop while developing. Once you get it working you can install it on a web server somewhere.
Setup Python
Open a terminal and see if python is already installed by typing:
python --version
If python isn’t installed install python. Pip is python’s package manager. We will need it to install the discord package. Make sure pip is installed, and if not, install pip.
Install the discord package using pip:
pip install discord.py
Write your bot code
Make a file called mybot.py (or whatever you want to call it) and fill it out with the following:
import asyncio import aiohttp import json import discord from discord import Game from discord.ext.commands import Bot # The command prefix that the bot will respond to BOT_PREFIX = ("!") # Your discord bot secret token. # If you don't have it saved, get this from https://discordapp.com/developers/applications/me TOKEN = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" bot = Bot(command_prefix=BOT_PREFIX) @bot.event async def on_ready(): await bot.change_presence(game=Game(name=BOT_PREFIX + " for commands")) print("Logged in as " + bot.user.name) @bot.command(name='bitcoin', description="Current price of bitcoin", brief="Bitcoin price", aliases=['btc'], pass_context=True) async def bitcoin(ctx): url = 'https://api.coindesk.com/v1/bpi/currentprice/BTC.json' async with aiohttp.ClientSession() as session: # Async HTTP request raw_response = await session.get(url) response = await raw_response.text() response = json.loads(response) await bot.say("Bitcoin price is: $" + response['bpi']['USD']['rate']) async def list_servers(): await bot.wait_until_ready() while not bot.is_closed: print("Current servers:") for server in bot.servers: print(server.name) await asyncio.sleep(600) if __name__ == "__main__": bot.loop.create_task(list_servers()) bot.run(TOKEN)
Run your code
If you are on the command line run your code like this:
python mybot.py
If you use an IDE, like Visual Studio Code, you can run it from there.
You should see in the output that the bot connects and logs in. You can then test your bot by issuing the command !bitcoin anywhere in your server. The bot should respond with the current price of bitcoin.
Additional Actions
The following are things that I did to make it easier to develop and deploy my bot:
- Use Microsoft Visual Studio Code (or some other IDE) to edit and debug your python project. You can run your bot in debug mode and set breakpoints so that when you hit certain commands you can step through line by line and see the data that your variables hold. I found Visual Studio Code to be easy to set up and get started with. I followed this tutorial, but here are the highlights:
- Install Visual Studio Code for your system.
- Use the built in package manager to install Microsoft’s python package.
- If you are using python environments, you will need to tell Visual Studio Code to use which environment to use. I used pyenv to create an environment which was stored in a folder called “env”. If you place this folder within the project directory then Visual Studio Code automatically discovers it.
- Use source control. I created a git repository using bitbucket. You could also use github or some other service. In addition to the normal benefits of source control, this allows me to easily deploy updated versions of my bot to the server. I develop and test the code on my local machine and then check in the changes to the repo. I cloned the repository on my server (an Amazon EC2 instance) so I simply have to log in to the server and pull the changes and re-run the bot.
- Deploy your bot to a server. Running your bot locally is great, but it only works while your computer is running. I have my bot running on an Amazon EC2 instance which was remarkably easy to get running. (MUCH easier than getting my home linux server installed and configured)